I wanted to connect my Z80/Z180 systems to Arduino UNO, because sometimes it is an easy path to integrate cheap sensors and analog devices. There are lots of sensors and stuff for Arduino and also much software to drive it. It is definitely not true 8-bit retro-style, but it works.
I thought it was easy. Just connect cross-wired TX/RX pins and a GND connection.
Nope.
After several hours of frustration, I finally found out that Arduino RX pin 0 and TX pin 1 is closely coupled to the USB port of the Arduino UNO, and apparently it is not really suitable for “general” serial communications.
To make it work, choose two different pins, like pin 2 and 3, and connect those to TX/RX on the Z80/Z180 serial port. In your sketch, use the library SoftwareSerial to define the serial interface on pin 2 and 3 – that did the trick for me.
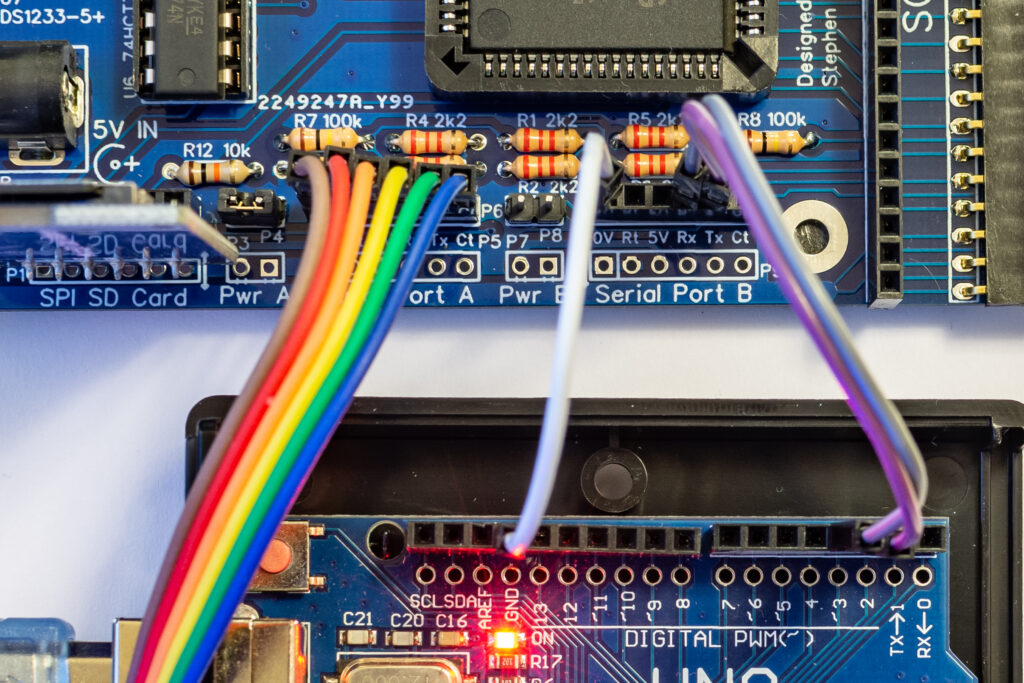
In the picture above it may be a little hard to see, but Arduino (in the bottom) pin 2 goes to TX and pin 3 goes to RX. GND goes to 0V.
By default the SC130 configures the baudrate to be 115200, which may be a little too high for software serial connection. I reduced speed to 9600, which is absolutely reliable. In CP/M 3 you can use the MODE command to easily change the baudrate (MODE COM1:9600,N,8,1
).
Turbo Pascal Example for CP/M
Note that the builtin “file” aux in Turbo Pascal maps to the AUX: device in CP/M, making it very easy to read/write the secondary serial port (COM1 on the SC130 in this case). Remember to run the MODE command in CP/M to set the correct baudrate for the port. This example assumes 9600 baud.
program echo;
var
msg: string[132];
begin
while true do begin
writeln(aux,'SC130 speaking…');
readln(aux, msg);
writeln(msg);
delay(2000);
end;
end.
Arduino Uno Sketch
I used pins 2 and 3 in this example, you are free to use any of the digital I/O pins for software serial communication.
#include <SoftwareSerial.h>
#define RX_PIN (2)
#define TX_PIN (3)
#define LED (13)
#define BUFSIZE (132)
#define CR (0x0D)
#define LF (0x0A)
#define BAUDRATE (9600)
#define EMPTY_STRING ""
SoftwareSerial auxSerial(RX_PIN, TX_PIN);
String buffer;
void setup() {
buffer.reserve(BUFSIZE);
buffer = EMPTY_STRING;
pinMode(LED, OUTPUT);
auxSerial.begin(BAUDRATE);
}
void loop() {
while (auxSerial.available() > 0) {
digitalWrite(LED, HIGH);
char ch = auxSerial.read();
if (ch == CR) {
auxSerial.println("Arduino says: " + buffer);
buffer = EMPTY_STRING;
} else {
if (ch != LF && buffer.length() < BUFSIZE-1) {
buffer = buffer + ch;
}
}
digitalWrite(LED, LOW);
}
}